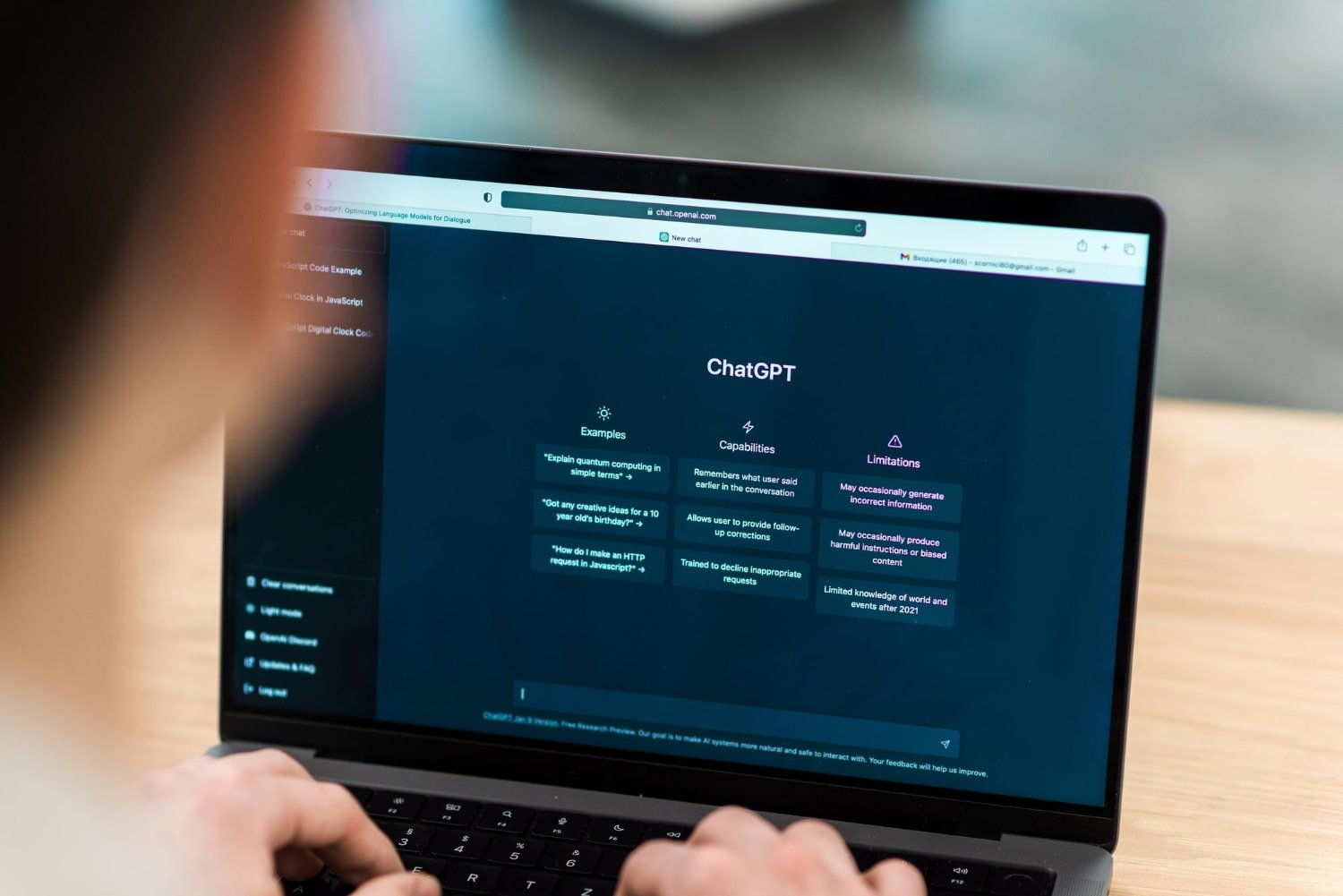
ChatGPT isn’t just a conversational AI—it’s rapidly emerging as an indispensable assistant for software developers. From generating boilerplate code and dissecting complex logic to crafting learning schedules for new programming languages, ChatGPT can augment your workflow with its array of powerful features. In this article, we’ll explore key ChatGPT functionalities—such as Search, Reason, and Explore GPTs—and illustrate how they can be harnessed through detailed examples and practical prompts.
1. ChatGPT Features Tailored for Developers
A. Search:
Instant Access to Up-to-Date Information
One of ChatGPT’s standout capabilities is its built-in search functionality, which enables developers to quickly fetch accurate summaries, comparisons, and documentation insights.
To use this feature, select the search option below the prompt input or use keywords like ‘Search or Scan internet’.
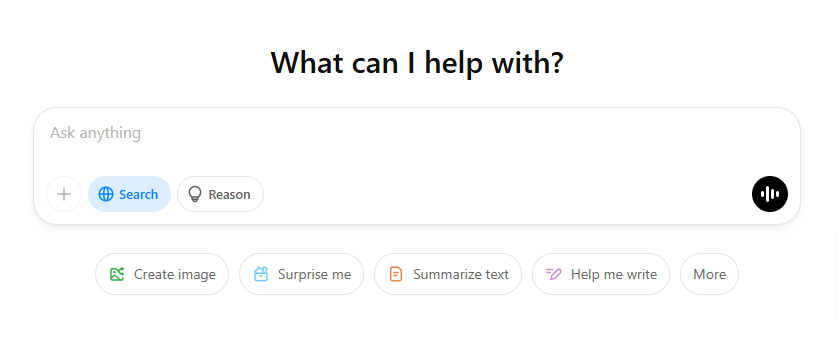
Example Prompt:
“Compare the advantages and limitations of Node.js vs. Deno for backend development.”
What You Get:
ChatGPT will compile a concise yet informative overview that covers performance aspects, security features, community support, and ecosystem maturity—saving you the time of sifting through multiple articles.
B. Reason:
Deep Dive into Code Analysis
ChatGPT’s Reason capability allows you to deconstruct and understand complex codebases. This feature is invaluable when dealing with legacy code or unfamiliar programming paradigms.
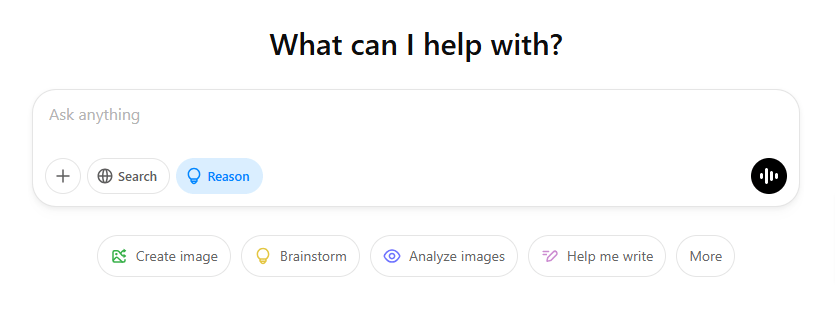
-
Example Scenario:
Imagine encountering a convoluted asynchronous function in JavaScript that uses callbacks and promises in an intricate way. You can prompt ChatGPT as follows:- Prompt:
“Explain what this JavaScript code does and how it handles asynchronous operations:
function fetchData(url, callback) {
fetch(url)
.then(response => response.json())
.then(data => callback(null, data))
.catch(error => callback(error, null));
}fetchData(‘https://api.example.com/data’, (err, data) => {
if (err) {
console.error(‘Error:’, err);
} else {
console.log(‘Data received:’, data);
}
});
Feedback:
ChatGPT will break down the code into logical parts: fetching data using the Fetch API, handling JSON conversion, error management, and invoking callbacks based on the outcome. This helps you grasp not only what the code does but also the rationale behind its structure.
C. Explore GPTs:
Accessing Custom Models for Specialized Tasks
ChatGPT’s Explore GPTs feature lets you tap into custom-built models tailored for specific coding needs. These models can be optimized for tasks like code summarization, automated refactoring, or even language-specific assistance.
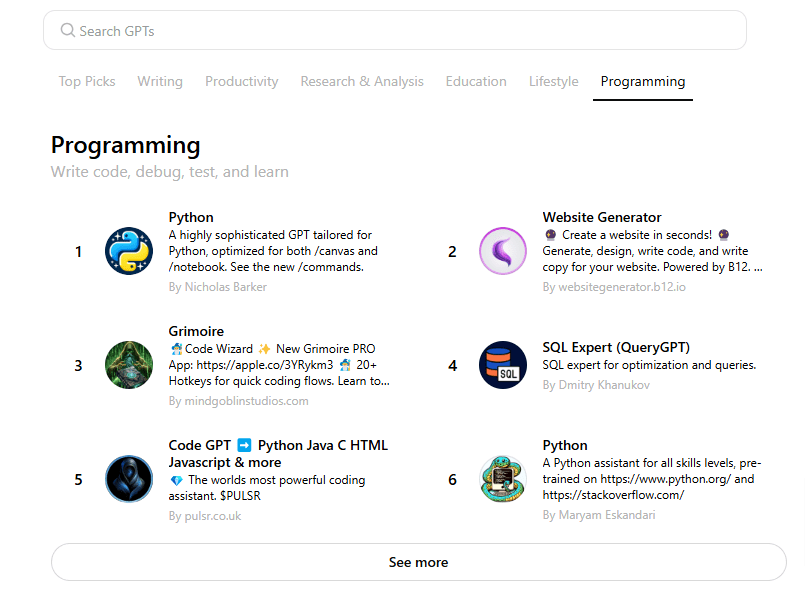
-
Example Use Case: Code Refactoring
Suppose you’re maintaining an aging Python codebase that has grown messy over time. You might use a custom GPT designed for refactoring:
- Prompt:
“Refactor the following Python function to improve readability and performance:
def process(data):
result = [] for i in range(len(data)):
if i % 2 == 0:
result.append(data[i] * 2)
else:
result.append(data[i] / 2)
return result
Feedback:
The specialized GPT could suggest using list comprehensions and slicing for cleaner code:
def process(data):
even = [x * 2 for x in data[::2]] odd = [x / 2 for x in data[1::2]] result = [] for e, o in zip(even, odd):
result.extend([e, o])
# Append the last element if data length is odd
if len(data) % 2 != 0:
result.append(even[-1])
return result
2. Practical Use Cases for Software Developers
A. Generating Boilerplate Code
Writing repetitive code is time-consuming. ChatGPT can automate this process, allowing you to focus on more challenging aspects of your project.
-
Example Prompt for a REST API Endpoint in Node.js:
“Generate boilerplate code for a Node.js Express server with a GET endpoint ‘/users’ that returns a JSON array of user objects.”
Expected Output:
const express = require(‘express’);
const app = express();
const port = 3000;// Sample user data
const users = [
{ id: 1, name: ‘Alice’ },
{ id: 2, name: ‘Bob’ },
];// GET endpoint to fetch users
app.get(‘/users’, (req, res) => {
res.json(users);
});app.listen(port, () => {
console.log(`Server running at http://localhost:${port}`);
});
This example demonstrates how ChatGPT can jump-start development by providing clean, ready-to-use code structures.
B. Implementing and Optimizing Algorithms
When you need a quick implementation of standard algorithms or want to optimize a current solution, ChatGPT can provide immediate, well-documented examples.
-
Example Prompt:
“Provide an optimized Python implementation of the QuickSort algorithm, including inline comments explaining each step.”
Expected Output:
def quicksort(arr):
“””
Sorts an array using the QuickSort algorithm.Args:
arr (list): List of elements to sort.Returns:
list: Sorted list.
“””
if len(arr) <= 1:
return arr # Base case: arrays with 0 or 1 element are already sorted
else:
pivot = arr[len(arr) // 2] # Partition the array into three parts
left = [x for x in arr if x < pivot] middle = [x for x in arr if x == pivot] right = [x for x in arr if x > pivot] # Recursively apply quicksort to partitions
return quicksort(left) + middle + quicksort(right)# Example usage:
unsorted_list = [3, 6, 8, 10, 1, 2, 1] print(“Sorted List:”, quicksort(unsorted_list))
This detailed, commented output makes it easier to understand and modify the algorithm according to your needs.
C. Learning Roadmap for Mastering Programming with ChatGPT
ChatGPT can serve as an excellent mentor in your programming journey, guiding you with structured learning roadmaps. Whether you’re a beginner learning Python or an experienced developer picking up a new framework, you can use ChatGPT to create a customized roadmap that includes foundational concepts, practical exercises, and milestone projects.
Example Prompt:
“I want to learn Python from scratch and become proficient in 3 months. Create a structured learning roadmap with key milestones, weekly goals, practical exercises, and project ideas.”
Python Learning Roadmap (3 Months)
🚀 Phase 1: Fundamentals & Core Syntax (Weeks 1-4)
📌 Goal: Build a strong foundation in Python syntax, logic, and problem-solving.
✅ Topics Covered:
- Python basics: variables, data types, operators
- Control structures: loops, if-else conditions
- Functions & modules
- Basic debugging techniques
🛠 Practical Exercises:
- Solve basic problems on platforms like LeetCode or CodeWars
- Implement simple programs like a temperature converter
📌 Milestone Project:
- Task: Build a command-line calculator that performs basic arithmetic operations.
⚙️ Phase 2: Intermediate Concepts & Real-World Applications (Weeks 5-8)
📌 Goal: Gain familiarity with object-oriented programming (OOP), data handling, and working with APIs.
✅ Topics Covered:
- Object-Oriented Programming (OOP) in Python
- File handling (reading/writing files)
- Data structures: lists, dictionaries, sets
- Working with APIs and HTTP requests
🛠 Practical Exercises:
- Implement a text file analyzer that counts word frequencies
- Use an API (e.g., OpenWeather API) to fetch and display weather data
📌 Milestone Project:
- Task: Develop a personal expense tracker that reads/writes data to a file and categorizes spending.
🖥️ Phase 3: Advanced Topics & Building Applications (Weeks 9-12)
📌 Goal: Learn how to develop scalable applications, handle concurrency, and deploy projects.
✅ Topics Covered:
- Asynchronous programming & threading
- Web development with Flask or FastAPI
- Database management with SQLite/PostgreSQL
- Testing & debugging strategies
- Deployment with Docker
🛠 Practical Exercises:
- Refactor previous projects to use asynchronous functions
- Build a REST API using Flask with basic CRUD operations
📌 Final Capstone Project:
- Task: Develop a full-stack web application (e.g., a to-do list app) using Flask, a database, and user authentication.
How ChatGPT Helps in Your Learning Journey
- 📖 Concept Explanations: Get instant answers for difficult programming concepts.
- 🔄 Practice & Feedback: Submit your code for review and receive improvement suggestions.
- 🛠 Project Guidance: Get help debugging and optimizing your milestone projects.
- 📅 Roadmap Adjustments: Ask ChatGPT to modify your roadmap based on your progress and goals.
Example Follow-Up Prompts:
“I’ve completed Phase 1 of my Python roadmap. What’s next?”
“Can you give me a more advanced project idea for Flask?”
D. Assisting with Code Reviews and Debugging
ChatGPT can help you perform preliminary code reviews, highlight potential issues, and suggest optimizations. You can input segments of your code with a prompt like:
- Example Prompt:
“Review the following Python function for potential improvements and error handling:
def calculate_discount(price, discount):
if discount > price:
return 0
return price – discount
Expected Feedback:
ChatGPT might suggest:
- Checking if
price
anddiscount
are of numeric types. - Handling negative values.
- Providing more meaningful error messages.
A revised version might look like:
def calculate_discount(price, discount):
# Ensure inputs are positive numbers
if not (isinstance(price, (int, float)) and isinstance(discount, (int, float))):
raise ValueError(“Price and discount must be numeric values.”)
if price < 0 or discount < 0:
raise ValueError(“Price and discount cannot be negative.”)
if discount > price:
return 0 # Cannot discount more than the price
return price – discount
This type of guided review aids in maintaining high code quality and reducing bugs before they escalate.
3. Conclusion
ChatGPT is evolving into a multifaceted tool for software developers, empowering you to:
- Quickly access and compare information with its Search feature,
- Break down complex code with its Reason capability,
- Customize your coding experience by exploring specialized GPTs, and
- Accelerate your learning and code quality with guided schedules and code reviews.
By integrating these functionalities into your daily workflow, you can streamline development processes, reduce debugging time, and continuously improve your coding skills. Whether you’re a seasoned developer or just starting your programming journey, ChatGPT offers a powerful, interactive platform to elevate your craft.
Embrace the future of AI-assisted development and explore the endless possibilities that ChatGPT brings to the software development lifecycle.